Projects Category: REGEX
- Home
- REGEX
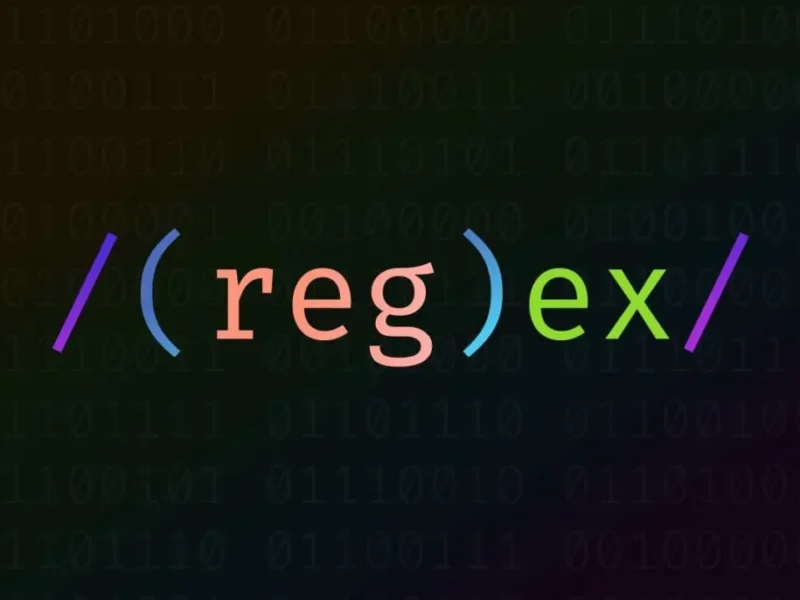
A comprehensive guide to advanced regular expressions for data mining and extraction
Introduction
In today’s data-driven world, the ability to efficiently extract structured information from unstructured text is invaluable. While many sophisticated NLP and machine learning tools exist for this purpose, regular expressions (regex) remain one of the most powerful and flexible tools in a data scientist’s toolkit. This blog explores advanced regex techniques implemented in the “Advance-Regex-For-Data-Mining-Extraction” project by Tejas K., demonstrating how carefully crafted patterns can transform raw text into actionable insights.
What Makes Regex Essential for Text Mining?
Regular expressions provide a concise, pattern-based approach to text processing that is:
- Language-agnostic: Works across programming languages and text processing tools
- Highly efficient: Once optimized, regex patterns can process large volumes of text quickly
- Precisely targeted: Allows extraction of exactly the information you need
- Flexible: Can be adapted to handle variations in text structure and format
Core Advanced Regex Techniques
Lookahead and Lookbehind Assertions
Lookahead (?=
) and lookbehind (?<=
) assertions are powerful techniques that allow matching patterns based on context without including that context in the match itself.
(?<=Price: \$)\d+\.\d{2}
This pattern matches a price value but only if it’s preceded by “Price: $”, without including “Price: $” in the match.
Non-Capturing Groups
When you need to group parts of a pattern but don’t need to extract that specific group:
(?:https?|ftp):\/\/[\w\.-]+\.[\w\.-]+
The ?:
tells the regex engine not to store the protocol match (http, https, or ftp), improving performance.
Named Capture Groups
Named capture groups make your regex more readable and the extracted data more easily accessible:
(?<date>\d{2}-\d{2}-\d{4}).*?(?<email>[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,})
Instead of working with numbered groups, you can now reference the extractions by name: date
and email
.
Balancing Groups for Nested Structures
The project implements sophisticated balancing groups for parsing nested structures like JSON or HTML:
\{(?<open>\{)|(?<-open>\})|[^{}]*\}(?(open)(?!))
This pattern matches properly nested curly braces, essential for parsing structured data formats.
Real-World Applications in the Project
1. Extracting Structured Information from Resumes
The project demonstrates how to parse unstructured resume text to extract:
Education: (?<education>(?:(?!Experience|Skills).)+)
Experience: (?<experience>(?:(?!Education|Skills).)+)
Skills: (?<skills>.+)
This pattern breaks a resume into logical sections, making it possible to analyze each component separately.
2. Mining Financial Data from Reports
Annual reports and financial statements contain valuable data that can be extracted with patterns like:
Revenue of \$(?<revenue>[\d,]+(?:\.\d+)?) million in (?<year>\d{4})
This extracts both the revenue figure and the corresponding year in a single operation.
3. Processing Log Files
The project includes patterns for parsing common log formats:
(?<ip>\d{1,3}\.\d{1,3}\.\d{1,3}\.\d{1,3}) - - \[(?<datetime>[^\]]+)\] "(?<request>[^"]*)" (?<status>\d+) (?<size>\d+)
This extracts IP addresses, timestamps, request details, status codes, and response sizes from standard HTTP logs.
Performance Optimization Techniques
1. Catastrophic Backtracking Prevention
The project implements strategies to avoid catastrophic backtracking, which can cause regex operations to hang:
# Instead of this (vulnerable to backtracking)
(\w+\s+){1,5}
# Use this (prevents backtracking issues)
(?:\w+\s+){1,5}?
2. Atomic Grouping
Atomic groups improve performance by preventing unnecessary backtracking:
(?>https?://[\w-]+(\.[\w-]+)+)
Once the atomic group matches, the regex engine doesn’t try alternative ways to match it.
3. Strategic Anchoring
Using anchors strategically improves performance by limiting where the regex engine needs to look:
^Subject: (.+)$
By anchoring to line start/end, the engine only attempts matches at line boundaries.
Implementation in Python
The project primarily uses Python’s re
module for implementation:
import re
def extract_structured_data(text):
pattern = r'Name: (?P<name>[\w\s]+)\s+Email: (?P<email>[^\s]+)\s+Phone: (?P<phone>[\d\-\(\)\s]+)'
match = re.search(pattern, text, re.MULTILINE)
if match:
return match.groupdict()
return None
For more complex operations, the project leverages the more powerful regex
module which supports advanced features like recursive patterns:
import regex
def extract_nested_structures(text):
pattern = r'\((?:[^()]++|(?R))*+\)' # Recursive pattern for nested parentheses
matches = regex.findall(pattern, text)
return matches
Case Study: Extracting Product Information from E-commerce Text
One compelling example from the project is extracting product details from unstructured e-commerce descriptions:
Product: Premium Bluetooth Headphones XC-400
SKU: BT-400-BLK
Price: $149.99
Available Colors: Black, Silver, Blue
Features: Noise Cancellation, 30-hour Battery, Water Resistant
Using this regex pattern:
Product: (?<product>.+?)[\r\n]+
SKU: (?<sku>[A-Z0-9\-]+)[\r\n]+
Price: \$(?<price>\d+\.\d{2})[\r\n]+
Available Colors: (?<colors>.+?)[\r\n]+
Features: (?<features>.+)
The code extracts a structured object:
{
"product": "Premium Bluetooth Headphones XC-400",
"sku": "BT-400-BLK",
"price": "149.99",
"colors": "Black, Silver, Blue",
"features": "Noise Cancellation, 30-hour Battery, Water Resistant"
}
Best Practices and Lessons Learned
The project emphasizes several best practices for regex-based data extraction:
- Test with diverse data: Ensure your patterns work with various text formats and edge cases
- Document complex patterns: Add comments explaining the logic behind complex regex
- Break complex patterns into components: Build and test incrementally
- Balance precision and flexibility: Overly specific patterns may break with slight text variations
- Consider preprocessing: Sometimes cleaning text before applying regex yields better results
Future Directions
The “Advance-Regex-For-Data-Mining-Extraction” project continues to evolve with plans to:
- Implement more domain-specific extraction patterns for legal, medical, and technical texts
- Create a pattern library organized by text type and extraction target
- Develop a visual pattern builder to make complex regex more accessible
- Benchmark performance against machine learning approaches for similar extraction tasks
Conclusion
Regular expressions remain a remarkably powerful tool for text mining and data extraction. The techniques demonstrated in this project show how advanced regex can transform unstructured text into structured, analyzable data with precision and efficiency. While newer technologies like NLP models and machine learning techniques offer alternative approaches, the flexibility, speed, and precision of well-crafted regex patterns ensure they’ll remain relevant for data mining tasks well into the future.
By mastering the advanced techniques outlined in this blog post, you’ll be well-equipped to tackle complex text mining challenges and extract meaningful insights from the vast sea of unstructured text data that surrounds us.
This blog post explores the techniques implemented in the Advance-Regex-For-Data-Mining-Extraction project by Tejas K.