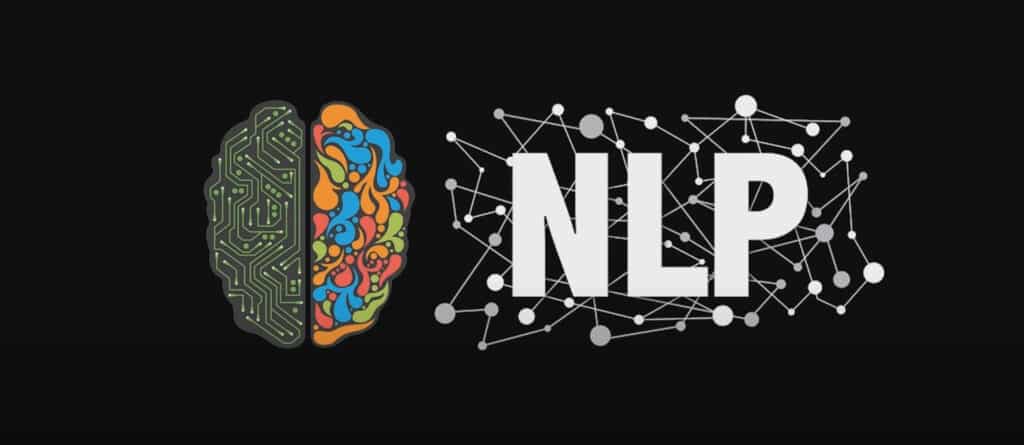
Leveraging Word2Vec: Practical Applications of Google’s 3 Billion Word Pre-trained Model
In the ever-evolving field of Natural Language Processing (NLP), word embeddings have revolutionized how machines understand human language. Among these technologies, Word2Vec stands as a foundational approach that transforms words into meaningful vector representations. This blog explores the practical implementation of Word2Vec using Google’s massive pre-trained model trained on approximately 3 billion words and phrases, demonstrating its versatility through diverse use cases.
Understanding Word2Vec: Beyond Simple Word Representation
Word2Vec, developed by researchers at Google, transforms words into numerical vectors where semantic relationships between words are preserved in vector space. Unlike traditional one-hot encoding methods, Word2Vec captures the contextual meaning of words, allowing machines to understand language nuances previously beyond their grasp.
Key Advantages of Word2Vec
- Semantic Relationships: Word2Vec captures semantic similarities between words, placing related concepts closer in vector space.
- Dimensionality Efficiency: While maintaining rich semantic information, Word2Vec typically uses only 300 dimensions per word (compared to vocabulary-sized vectors in one-hot encoding).
- Arithmetic Operations on Words: Perhaps most fascinating is Word2Vec’s ability to perform meaningful arithmetic with words. The classic example
king - man + woman ≈ queen
demonstrates how these vectors encode gender, royalty, and other semantic concepts. - Transfer Learning Capability: Pre-trained embeddings allow models to benefit from knowledge learned on massive text corpora without requiring extensive training data.
- Language Agnosticism: The core techniques work across languages, making it valuable for multilingual applications.
- Handling Out-of-Vocabulary Words: With techniques like subword embeddings, Word2Vec approaches can handle previously unseen words.
Exploring the Practical Implementation
Looking at the implementation repository, we can see how Google’s pre-trained model is leveraged through the gensim
library. Let’s explore some of the practical applications and extend them further.
Word Similarity and Relationships
The repository demonstrates finding similar words—a fundamental application of Word2Vec. For example, finding words most similar to “intelligent” reveals words like “smart,” “brilliant,” and “clever.” This capability forms the foundation for many downstream applications, from recommendation systems to semantic search.
model.most_similar("intelligent")
Analogical Reasoning
Word2Vec’s ability to perform word arithmetic allows for solving analogies:
model.most_similar(positive=['woman', 'king'], negative=['man'])
This returns “queen” as the top result, demonstrating the model’s understanding of gender relationships combined with royal status.
Advanced Use Cases for Google’s Pre-trained Model
Let’s explore additional applications beyond those covered in the repository, leveraging the power of Google’s 3-billion-word pre-trained embeddings:
1. Document Classification and Clustering
By averaging Word2Vec vectors for all words in a document, we can create document vectors for classification or clustering:
def document_vector(doc):
words = doc.lower().split()
word_vectors = [model[word] for word in words if word in model]
return np.mean(word_vectors, axis=0) if word_vectors else np.zeros(model.vector_size)
# Example documents
documents = [
"Artificial intelligence is transforming healthcare systems globally",
"Machine learning algorithms help diagnose diseases early",
"The stock market fluctuated significantly last quarter",
"Investors are concerned about economic indicators"
]
# Create document vectors
doc_vectors = [document_vector(doc) for doc in documents]
# Cluster documents
from sklearn.cluster import KMeans
kmeans = KMeans(n_clusters=2)
clusters = kmeans.fit_predict(doc_vectors)
This approach can group documents by topic without explicit topic modeling.
2. Sentiment Analysis Enhancement
Word2Vec can improve sentiment analysis by accounting for semantic relationships:
def sentiment_score(text, positive_words, negative_words):
words = text.lower().split()
score = 0
for word in words:
if word in model:
# Calculate similarity to positive and negative word sets
pos_similarity = np.mean([model.similarity(word, pos) for pos in positive_words if pos in model])
neg_similarity = np.mean([model.similarity(word, neg) for neg in negative_words if neg in model])
score += (pos_similarity - neg_similarity)
return score / max(len(words), 1) # Normalize by text length
# Example usage
positive_words = ["excellent", "amazing", "wonderful", "great"]
negative_words = ["terrible", "awful", "horrible", "bad"]
texts = [
"The product exceeded my expectations and works flawlessly.",
"This was a complete waste of money and time."
]
for text in texts:
print(f"Text: {text}")
print(f"Sentiment score: {sentiment_score(text, positive_words, negative_words):.4f}")
This method can detect sentiment in texts containing words not explicitly in our sentiment lexicons.
3. Named Entity Recognition Support
Word2Vec embeddings can enhance named entity recognition by providing semantic context:
def is_likely_organization(word, context_words):
org_indicators = ["company", "corporation", "organization", "enterprise"]
if word not in model:
return False
# Check similarity to organization indicators
org_similarity = np.mean([model.similarity(word, org) for org in org_indicators if org in model])
# Check if context suggests an organization
context_similarity = 0
if context_words:
context_vectors = [model[w] for w in context_words if w in model]
if context_vectors:
context_vector = np.mean(context_vectors, axis=0)
for org in org_indicators:
if org in model:
context_similarity += cosine_similarity([model[org]], [context_vector])[0][0]
context_similarity /= len(org_indicators)
return (org_similarity > 0.3) or (context_similarity > 0.4)
4. Concept Expansion and Exploration
Word2Vec can help expand topic-related terms for content creation or research:
def explore_concept(seed_terms, depth=2, breadth=5):
"""Explore related concepts starting from seed terms."""
all_terms = set(seed_terms)
current_terms = seed_terms
for d in range(depth):
next_level = []
for term in current_terms:
if term in model:
similar_terms = [word for word, _ in model.most_similar(term, topn=breadth)]
next_level.extend(similar_terms)
next_level = list(set(next_level) - all_terms) # Remove duplicates
all_terms.update(next_level)
current_terms = next_level
return all_terms
# Example: Explore AI-related concepts
ai_concepts = explore_concept(["artificial_intelligence", "machine_learning"], depth=2, breadth=7)
This function can help researchers explore interconnected concepts or content creators develop comprehensive topic coverage.
5. Translation Assistance
While not a complete translation system, Word2Vec can help with cross-language word mapping:
def find_translation_candidates(word, source_model, target_model, bridge_words):
"""Find possible translations using bridge words known in both languages."""
if word not in source_model:
return []
candidates = {}
for bridge in bridge_words:
if bridge in source_model and bridge in target_model:
# Find words similar to our word in source language
source_similar = [w for w, _ in source_model.most_similar(word, topn=10)]
# For each similar word, find corresponding words in target language
for s_word in source_similar:
if s_word in source_model:
# Use the bridge word to find target language equivalents
target_similar = [w for w, _ in target_model.most_similar(bridge, topn=20)]
for t_word in target_similar:
candidates[t_word] = candidates.get(t_word, 0) + 1
# Return candidates sorted by frequency
return sorted(candidates.items(), key=lambda x: x[1], reverse=True)
Research Implications and Future Directions
The Google pre-trained Word2Vec model’s 3 billion word training corpus offers several research advantages:
- Robust Representation: The massive training corpus ensures stable, noise-resistant word representations capturing subtle semantic relationships.
- Knowledge Transfer: Pre-trained embeddings transfer knowledge from vast text collections to specialized domains with limited training data.
- Cross-domain Applications: Word2Vec’s language agnosticism allows transferring knowledge across domains—using knowledge from general corpora for specialized applications like medical text analysis.
- Foundation for Advanced Architectures: While newer models like BERT and GPT have emerged, Word2Vec remains relevant as a lightweight alternative and serves as the conceptual foundation for these more complex architectures.
- Interpretability: Unlike black-box transformers, Word2Vec representations are more interpretable through techniques like principal component analysis of word vectors.
Challenges and Limitations
Despite its advantages, researchers should be aware of Word2Vec’s limitations:
- Context Insensitivity: Each word has exactly one vector, regardless of context (unlike BERT’s contextual embeddings).
- Training Corpus Bias: Embeddings inherit biases present in the training corpus, potentially perpetuating stereotypes.
- Rare Word Problem: Words appearing infrequently in the training corpus have less reliable representations.
- Computational Requirements: While more efficient than newer transformer models, loading Google’s pre-trained vectors still requires significant memory.
Conclusion
Google’s pre-trained Word2Vec model trained on 3 billion words offers a powerful foundation for numerous NLP applications. From semantic search to document classification, sentiment analysis to concept exploration, these word embeddings continue to provide value despite newer architectures.
The practical implementations explored in this blog demonstrate how a single pre-trained model can address diverse language understanding challenges without extensive additional training. As NLP research advances, Word2Vec remains relevant as both a standalone solution for many applications and a conceptual building block for understanding more complex embedding approaches.
For researchers and practitioners working with limited computational resources or seeking interpretable word representations, Google’s pre-trained Word2Vec model remains an invaluable tool in the NLP toolkit.